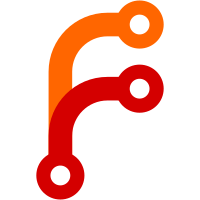
the busName and interfacePath types transparently cast down to a string, (but not the other way around) so they are a perfect upgrade to type-safety. The only downside to them is that templates can often get them wrong. std.conv.to will return with a cast(T) prefix, which could slightly break existing code. I think the type-safety advantages are outweighing this corner case though. The current API has been fully upgraded and examples still run and work.
27 lines
788 B
D
27 lines
788 B
D
import std.stdio;
|
|
import ddbus;
|
|
|
|
void testServe(Connection conn) {
|
|
auto router = new MessageRouter();
|
|
MessagePattern patt = MessagePattern(ObjectPath("/root"), interfaceName("ca.thume.test"), "test");
|
|
router.setHandler!(int,int)(patt,(int par) {
|
|
writeln("Called with ", par);
|
|
return par;
|
|
});
|
|
patt = MessagePattern(ObjectPath("/signaler"), interfaceName("ca.thume.test"), "signal",true);
|
|
router.setHandler!(void,int)(patt,(int par) {
|
|
writeln("Signalled with ", par);
|
|
});
|
|
registerRouter(conn, router);
|
|
writeln("Getting name...");
|
|
bool gotem = requestName(conn, busName("ca.thume.ddbus.test"));
|
|
writeln("Got name: ",gotem);
|
|
simpleMainLoop(conn);
|
|
}
|
|
|
|
void main() {
|
|
Connection conn = connectToBus();
|
|
testServe(conn);
|
|
writeln("It worked!");
|
|
}
|