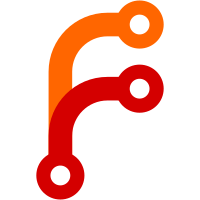
After experiments with loading the whole file at once, and with decoding and parsing in separate thread, lazy reader turned to be the fastest/least memory intensive solution. Characters are now decoded in small batches. This improved parsing speed by ~20%. No global state anymore. Anchors are now zero terminated strings and TagDirectives are a simple array. Event structure was changed to prevent size increase. Minor fixes and improvements.
232 lines
9 KiB
HTML
232 lines
9 KiB
HTML
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
|
|
"http://www.w3.org/TR/html4/strict.dtd">
|
|
|
|
<html lang='en'>
|
|
<head>
|
|
<meta http-equiv="content-type" content="text/html; charset=utf-8" >
|
|
<title>dyaml.dumper - D:YAML 0.3 API documentation</title>
|
|
<link rel="stylesheet" type="text/css" href="css/style.css">
|
|
</head>
|
|
|
|
<body><div id="top">
|
|
<div id="header">
|
|
<img id="logo" alt="D:YAML logo" src="images/logo.png"><a id="main-heading" href="index.html">D:YAML 0.3 API documentation</a>
|
|
</div>
|
|
</div>
|
|
|
|
<div id="navigation">
|
|
<div class="navblock">
|
|
<div id="toctop">
|
|
<ul><li><a href="../index.html">Documentation home</a></li>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
<div class="navblock">
|
|
<ul><li><a href="index.html">Main page</a></li>
|
|
<li><a href="dyaml.constructor.html">dyaml.constructor</a></li>
|
|
<li><a href="dyaml.dumper.html">dyaml.dumper</a></li>
|
|
<li><a href="dyaml.encoding.html">dyaml.encoding</a></li>
|
|
<li><a href="dyaml.exception.html">dyaml.exception</a></li>
|
|
<li><a href="dyaml.linebreak.html">dyaml.linebreak</a></li>
|
|
<li><a href="dyaml.loader.html">dyaml.loader</a></li>
|
|
<li><a href="dyaml.node.html">dyaml.node</a></li>
|
|
<li><a href="dyaml.representer.html">dyaml.representer</a></li>
|
|
<li><a href="dyaml.resolver.html">dyaml.resolver</a></li>
|
|
<li><a href="dyaml.style.html">dyaml.style</a></li>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
|
|
<div id="content">
|
|
<h1>dyaml.dumper</h1>
|
|
<!-- Generated by Ddoc from dyaml/dumper.d -->
|
|
<p>YAML dumper.
|
|
</p>
|
|
<p>Code based on <a href="http://www.pyyaml.org">PyYAML</a>.</p>
|
|
|
|
<dl><dt class="d_decl">struct <a name="Dumper"></a><span class="ddoc_psymbol">Dumper</span>;
|
|
</dt>
|
|
<dd><p>Dumps YAML documents to files or streams.
|
|
</p>
|
|
<p>User specified Representer and/or Resolver can be used to support new
|
|
tags / data types.
|
|
<br>
|
|
|
|
Setters are provided to affect output details (style, encoding, etc.).
|
|
|
|
</p>
|
|
<b>Examples:</b><div class="pbr">Write to a file:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> node = Node([1, 2, 3, 4, 5]);
|
|
<span class="d_psymbol">Dumper</span>(<span class="d_string">"file.yaml"</span>).dump(node);
|
|
</pre>
|
|
|
|
Write multiple YAML documents to a file:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> node1 = Node([1, 2, 3, 4, 5]);
|
|
<span class="d_keyword">auto</span> node2 = Node(<span class="d_string">"This document contains only one string"</span>);
|
|
<span class="d_psymbol">Dumper</span>(<span class="d_string">"file.yaml"</span>).dump(node1, node2);
|
|
|
|
<span class="d_comment">//Or with an array:
|
|
</span> <span class="d_comment">//Dumper("file.yaml").dump([node1, node2]);
|
|
</span>
|
|
|
|
</pre>
|
|
|
|
Write to memory:
|
|
<pre class="d_code"> <span class="d_keyword">import</span> std.stream;
|
|
<span class="d_keyword">auto</span> stream = <span class="d_keyword">new</span> MemoryStream();
|
|
<span class="d_keyword">auto</span> node = Node([1, 2, 3, 4, 5]);
|
|
<span class="d_psymbol">Dumper</span>(stream).dump(node);
|
|
</pre>
|
|
|
|
Use a custom representer/resolver to support custom data types and/or implicit tags:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> node = Node([1, 2, 3, 4, 5]);
|
|
<span class="d_keyword">auto</span> representer = <span class="d_keyword">new</span> Representer();
|
|
<span class="d_keyword">auto</span> resolver = <span class="d_keyword">new</span> Resolver();
|
|
|
|
<span class="d_comment">//Add representer functions / resolver expressions here...
|
|
</span>
|
|
<span class="d_keyword">auto</span> dumper = <span class="d_psymbol">Dumper</span>(<span class="d_string">"file.yaml"</span>);
|
|
dumper.representer = representer;
|
|
dumper.resolver = resolver;
|
|
dumper.dump(node);
|
|
</pre>
|
|
</div>
|
|
|
|
<dl><dt class="d_decl">this(string <b>filename</b>);
|
|
</dt>
|
|
<dd><p>Construct a Dumper writing to a file.
|
|
</p>
|
|
<b>Parameters:</b><div class="pbr"><table class=parms><tr><td valign=top>string <b>filename</b></td>
|
|
<td valign=top>File name to write to.</td></tr>
|
|
</table></div>
|
|
<b>Throws:</b><div class="pbr">YAMLException if the file can not be dumped to (e.g. cannot be opened).</div>
|
|
|
|
</dd>
|
|
<dt class="d_decl">this(Stream <b>stream</b>);
|
|
</dt>
|
|
<dd><p>Construct a Dumper writing to a stream. This is useful to e.g. write to memory.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="name"></a><span class="ddoc_psymbol">name</span>(string <a name="name"></a><span class="ddoc_psymbol">name</span>);
|
|
</dt>
|
|
<dd><p>Set stream name. Used in debugging messages.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="resolver"></a><span class="ddoc_psymbol">resolver</span>(Resolver <a name="resolver"></a><span class="ddoc_psymbol">resolver</span>);
|
|
</dt>
|
|
<dd><p>Specify custom Resolver to use.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="representer"></a><span class="ddoc_psymbol">representer</span>(Representer <a name="representer"></a><span class="ddoc_psymbol">representer</span>);
|
|
</dt>
|
|
<dd><p>Specify custom Representer to use.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="canonical"></a><span class="ddoc_psymbol">canonical</span>(bool <a name="canonical"></a><span class="ddoc_psymbol">canonical</span>);
|
|
</dt>
|
|
<dd><p>Write scalars in canonical form?</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="indent"></a><span class="ddoc_psymbol">indent</span>(uint <a name="indent"></a><span class="ddoc_psymbol">indent</span>);
|
|
</dt>
|
|
<dd><p>Set indentation width. 2 by default. Must not be zero.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="textWidth"></a><span class="ddoc_psymbol">textWidth</span>(uint <b>width</b>);
|
|
</dt>
|
|
<dd><p>Set preferred text width.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="lineBreak"></a><span class="ddoc_psymbol">lineBreak</span>(LineBreak <a name="lineBreak"></a><span class="ddoc_psymbol">lineBreak</span>);
|
|
</dt>
|
|
<dd><p>Set line break to use. Unix by default.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="encoding"></a><span class="ddoc_psymbol">encoding</span>(Encoding <a name="encoding"></a><span class="ddoc_psymbol">encoding</span>);
|
|
</dt>
|
|
<dd><p>Set character encoding to use. UTF-8 by default.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="explicitStart"></a><span class="ddoc_psymbol">explicitStart</span>(bool <b>explicit</b>);
|
|
</dt>
|
|
<dd><p>Always explicitly write document start?</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="explicitEnd"></a><span class="ddoc_psymbol">explicitEnd</span>(bool <b>explicit</b>);
|
|
</dt>
|
|
<dd><p>Always explicitly write document end?</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="YAMLVersion"></a><span class="ddoc_psymbol">YAMLVersion</span>(string <a name="YAMLVersion"></a><span class="ddoc_psymbol">YAMLVersion</span>);
|
|
</dt>
|
|
<dd><p>Specify YAML version string. "1.1" by default.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="tagDirectives"></a><span class="ddoc_psymbol">tagDirectives</span>(string[string] <b>tags</b>);
|
|
</dt>
|
|
<dd><p>Specify tag directives.
|
|
</p>
|
|
<p>A tag directive specifies a shorthand notation for specifying tags.
|
|
Each tag directive associates a handle with a prefix. This allows for
|
|
compact tag notation.
|
|
<br>
|
|
|
|
Each handle specified MUST start and end with a '!' character
|
|
(a single character "!" handle is allowed as well).
|
|
<br>
|
|
|
|
Only alphanumeric characters, '-', and '_' may be used in handles.
|
|
<br>
|
|
|
|
Each prefix MUST not be empty.
|
|
<br>
|
|
|
|
The "!!" handle is used for default YAML tags with prefix
|
|
"tag:yaml.org,2002:". This can be overridden.
|
|
|
|
</p>
|
|
<b>Parameters:</b><div class="pbr"><table class=parms><tr><td valign=top>string[string] <b>tags</b></td>
|
|
<td valign=top>Tag directives (keys are handles, values are prefixes).</td></tr>
|
|
</table></div>
|
|
<p><b>Example:</b><br>
|
|
<pre class="d_code"> Dumper dumper = Dumper(<span class="d_string">"file.yaml"</span>);
|
|
string[string] directives;
|
|
directives[<span class="d_string">"!short!"</span>] = <span class="d_string">"tag:long.org,2011:"</span>;
|
|
<span class="d_comment">//This will emit tags starting with "tag:long.org,2011"
|
|
</span> <span class="d_comment">//with a "!short!" prefix instead.
|
|
</span> dumper.<span class="d_psymbol">tagDirectives</span>(directives);
|
|
dumper.dump(Node(<span class="d_string">"foo"</span>));
|
|
</pre>
|
|
</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">void <a name="dump"></a><span class="ddoc_psymbol">dump</span>(Node[] <b>documents</b>...);
|
|
</dt>
|
|
<dd><p>Dump one or more YAML documents to the file/stream.
|
|
</p>
|
|
<p>Note that while you can call <a name="dump"></a><span class="ddoc_psymbol">dump</span>() multiple times on the same
|
|
dumper, you will end up writing multiple YAML "files" to the same
|
|
file/stream.
|
|
|
|
</p>
|
|
<b>Parameters:</b><div class="pbr"><table class=parms><tr><td valign=top>Node[] <b>documents</b></td>
|
|
<td valign=top>Documents to dump (root nodes of the documents).</td></tr>
|
|
</table></div>
|
|
<b>Throws:</b><div class="pbr">YAMLException on error (e.g. invalid nodes,
|
|
unable to write to file/stream).</div>
|
|
|
|
</dd>
|
|
</dl>
|
|
</dd>
|
|
</dl>
|
|
|
|
</div>
|
|
|
|
<div id="copyright">
|
|
Copyright © Ferdinand Majerech 2011. Based on <a href="http://www.pyyaml.org">PyYAML</a> by Kirill Simonov. |
|
|
Page generated by Autodoc and <a href="http://www.digitalmars.com/d/2.0/ddoc.html">Ddoc</a>.
|
|
</div>
|
|
|
|
</body>
|
|
</html>
|