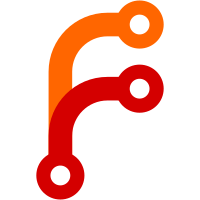
After experiments with loading the whole file at once, and with decoding and parsing in separate thread, lazy reader turned to be the fastest/least memory intensive solution. Characters are now decoded in small batches. This improved parsing speed by ~20%. No global state anymore. Anchors are now zero terminated strings and TagDirectives are a simple array. Event structure was changed to prevent size increase. Minor fixes and improvements.
198 lines
7.3 KiB
HTML
198 lines
7.3 KiB
HTML
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
|
|
"http://www.w3.org/TR/html4/strict.dtd">
|
|
|
|
<html lang='en'>
|
|
<head>
|
|
<meta http-equiv="content-type" content="text/html; charset=utf-8" >
|
|
<title>dyaml.loader - D:YAML 0.3 API documentation</title>
|
|
<link rel="stylesheet" type="text/css" href="css/style.css">
|
|
</head>
|
|
|
|
<body><div id="top">
|
|
<div id="header">
|
|
<img id="logo" alt="D:YAML logo" src="images/logo.png"><a id="main-heading" href="index.html">D:YAML 0.3 API documentation</a>
|
|
</div>
|
|
</div>
|
|
|
|
<div id="navigation">
|
|
<div class="navblock">
|
|
<div id="toctop">
|
|
<ul><li><a href="../index.html">Documentation home</a></li>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
<div class="navblock">
|
|
<ul><li><a href="index.html">Main page</a></li>
|
|
<li><a href="dyaml.constructor.html">dyaml.constructor</a></li>
|
|
<li><a href="dyaml.dumper.html">dyaml.dumper</a></li>
|
|
<li><a href="dyaml.encoding.html">dyaml.encoding</a></li>
|
|
<li><a href="dyaml.exception.html">dyaml.exception</a></li>
|
|
<li><a href="dyaml.linebreak.html">dyaml.linebreak</a></li>
|
|
<li><a href="dyaml.loader.html">dyaml.loader</a></li>
|
|
<li><a href="dyaml.node.html">dyaml.node</a></li>
|
|
<li><a href="dyaml.representer.html">dyaml.representer</a></li>
|
|
<li><a href="dyaml.resolver.html">dyaml.resolver</a></li>
|
|
<li><a href="dyaml.style.html">dyaml.style</a></li>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
|
|
<div id="content">
|
|
<h1>dyaml.loader</h1>
|
|
<!-- Generated by Ddoc from dyaml/loader.d -->
|
|
<p>Class used to load YAML documents.</p>
|
|
|
|
<dl><dt class="d_decl">struct <a name="Loader"></a><span class="ddoc_psymbol">Loader</span>;
|
|
</dt>
|
|
<dd><p>Loads YAML documents from files or streams.
|
|
</p>
|
|
<p>User specified Constructor and/or Resolver can be used to support new
|
|
tags / data types.
|
|
|
|
</p>
|
|
<b>Examples:</b><div class="pbr">Load single YAML document from a file:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> rootNode = <span class="d_psymbol">Loader</span>(<span class="d_string">"file.yaml"</span>).load();
|
|
...
|
|
</pre>
|
|
|
|
Load all YAML documents from a file:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> nodes = <span class="d_psymbol">Loader</span>(<span class="d_string">"file.yaml"</span>).loadAll();
|
|
...
|
|
</pre>
|
|
|
|
Iterate over YAML documents in a file, lazily loading them:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> loader = <span class="d_psymbol">Loader</span>(<span class="d_string">"file.yaml"</span>);
|
|
|
|
<span class="d_keyword">foreach</span>(<span class="d_keyword">ref</span> node; loader)
|
|
{
|
|
...
|
|
}
|
|
</pre>
|
|
|
|
Load YAML from memory:
|
|
<pre class="d_code"> <span class="d_keyword">import</span> std.stream;
|
|
<span class="d_keyword">import</span> std.stdio;
|
|
|
|
string yaml_input = <span class="d_string">"red: '#ff0000'\n"</span>
|
|
<span class="d_string">"green: '#00ff00'\n"</span>
|
|
<span class="d_string">"blue: '#0000ff'"</span>;
|
|
|
|
<span class="d_keyword">auto</span> colors = <span class="d_psymbol">Loader</span>(<span class="d_keyword">new</span> MemoryStream(<span class="d_keyword">cast</span>(<span class="d_keyword">char</span>[])yaml_input)).load();
|
|
|
|
<span class="d_keyword">foreach</span>(string color, string value; colors)
|
|
{
|
|
writeln(color, <span class="d_string">" is "</span>, value, <span class="d_string">" in HTML/CSS"</span>);
|
|
}
|
|
</pre>
|
|
|
|
Use a custom constructor/resolver to support custom data types and/or implicit tags:
|
|
<pre class="d_code"> <span class="d_keyword">auto</span> constructor = <span class="d_keyword">new</span> Constructor();
|
|
<span class="d_keyword">auto</span> resolver = <span class="d_keyword">new</span> Resolver();
|
|
|
|
<span class="d_comment">//Add constructor functions / resolver expressions here...
|
|
</span>
|
|
<span class="d_keyword">auto</span> loader = <span class="d_psymbol">Loader</span>(<span class="d_string">"file.yaml"</span>);
|
|
loader.constructor = constructor;
|
|
loader.resolver = resolver;
|
|
<span class="d_keyword">auto</span> rootNode = loader.load(node);
|
|
</pre>
|
|
</div>
|
|
|
|
<dl><dt class="d_decl">this(string <b>filename</b>);
|
|
</dt>
|
|
<dd><p>Construct a Loader to load YAML from a file.
|
|
</p>
|
|
<b>Parameters:</b><div class="pbr"><table class=parms><tr><td valign=top>string <b>filename</b></td>
|
|
<td valign=top>Name of the file to load from.</td></tr>
|
|
</table></div>
|
|
<b>Throws:</b><div class="pbr">YAMLException if the file could not be opened or read.</div>
|
|
|
|
</dd>
|
|
<dt class="d_decl">this(Stream <b>stream</b>);
|
|
</dt>
|
|
<dd><p>Construct a Loader to load YAML from a stream.
|
|
</p>
|
|
<b>Parameters:</b><div class="pbr"><table class=parms><tr><td valign=top>Stream <b>stream</b></td>
|
|
<td valign=top>Stream to read from. Must be readable and seekable.</td></tr>
|
|
</table></div>
|
|
<b>Throws:</b><div class="pbr">YAMLException if <b>stream</b> could not be read.</div>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="name"></a><span class="ddoc_psymbol">name</span>(string <a name="name"></a><span class="ddoc_psymbol">name</span>);
|
|
</dt>
|
|
<dd><p>Set stream name. Used in debugging messages.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="resolver"></a><span class="ddoc_psymbol">resolver</span>(Resolver <a name="resolver"></a><span class="ddoc_psymbol">resolver</span>);
|
|
</dt>
|
|
<dd><p>Specify custom Resolver to use.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">@property void <a name="constructor"></a><span class="ddoc_psymbol">constructor</span>(Constructor <a name="constructor"></a><span class="ddoc_psymbol">constructor</span>);
|
|
</dt>
|
|
<dd><p>Specify custom Constructor to use.</p>
|
|
|
|
</dd>
|
|
<dt class="d_decl">Node <a name="load"></a><span class="ddoc_psymbol">load</span>();
|
|
</dt>
|
|
<dd><p>Load single YAML document.
|
|
</p>
|
|
<p>If none or more than one YAML document is found, this throws a YAMLException.
|
|
<br>
|
|
|
|
This can only be called once; this is enforced by contract.
|
|
|
|
</p>
|
|
<b>Returns:</b><div class="pbr">Root node of the document.
|
|
|
|
</div>
|
|
<b>Throws:</b><div class="pbr">YAMLException if there wasn't exactly one document
|
|
or on a YAML parsing error.</div>
|
|
|
|
</dd>
|
|
<dt class="d_decl">Node[] <a name="loadAll"></a><span class="ddoc_psymbol">loadAll</span>();
|
|
</dt>
|
|
<dd><p>Load all YAML documents.
|
|
</p>
|
|
<p>This is just a shortcut that iterates over all documents and returns
|
|
them all at once. Calling <a name="loadAll"></a><span class="ddoc_psymbol">loadAll</span> after iterating over the node or
|
|
vice versa will not return any documents, as they have all been parsed
|
|
already.
|
|
<br>
|
|
|
|
This can only be called once; this is enforced by contract.
|
|
|
|
</p>
|
|
<b>Returns:</b><div class="pbr">Array of root nodes of all documents in the file/stream.
|
|
|
|
</div>
|
|
<b>Throws:</b><div class="pbr">YAMLException on a parsing error.</div>
|
|
|
|
</dd>
|
|
<dt class="d_decl">int <a name="opApply"></a><span class="ddoc_psymbol">opApply</span>(int delegate(ref Node) <b>dg</b>);
|
|
</dt>
|
|
<dd><p>Foreach over YAML documents.
|
|
</p>
|
|
<p>Parses documents lazily, when they are needed.
|
|
<br>
|
|
|
|
Foreach over a Loader can only be used once; this is enforced by contract.
|
|
|
|
</p>
|
|
<b>Throws:</b><div class="pbr">YAMLException on a parsing error.</div>
|
|
|
|
</dd>
|
|
</dl>
|
|
</dd>
|
|
</dl>
|
|
|
|
</div>
|
|
|
|
<div id="copyright">
|
|
Copyright © Ferdinand Majerech 2011. Based on <a href="http://www.pyyaml.org">PyYAML</a> by Kirill Simonov. |
|
|
Page generated by Autodoc and <a href="http://www.digitalmars.com/d/2.0/ddoc.html">Ddoc</a>.
|
|
</div>
|
|
|
|
</body>
|
|
</html>
|